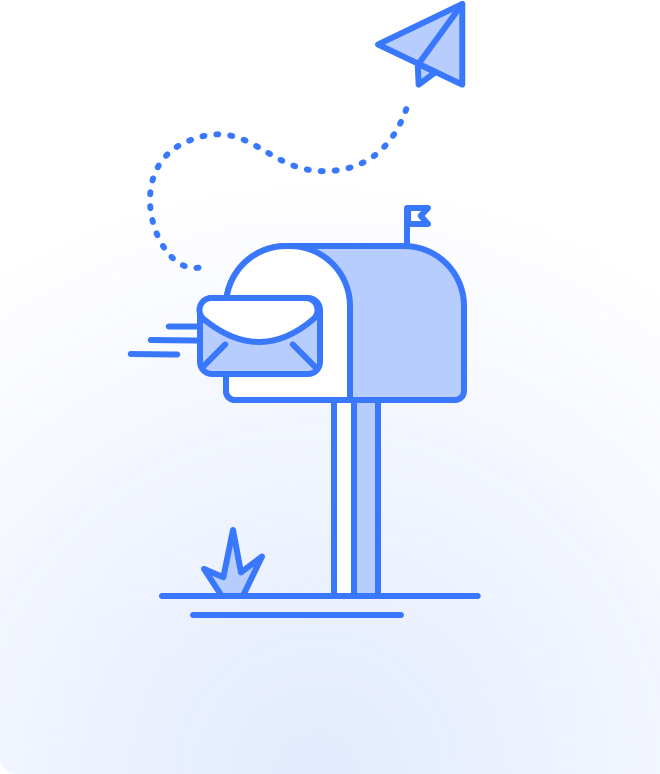
Welcome to the AI Rephrase Implementation Guide for iOS, your key to integrating the remarkable capabilities of AI Rephrase into your iOS applications. If you’re looking to enhance the clarity of communication, add a touch of personality to your messaging, or simply explore the potential of this cutting-edge technology, you’ve come to the right place.
With QuickBlox, you can easily create AI-powered chats in iOS apps. Whether you’re building an iOS chat app from scratch or using our convenient iOS UI Kit, the QuickBlox AI Library empowers you to seamlessly integrate AI rephrasing capabilities using the QBAIRephrase Swift package.
QuickBlox is dedicated to creating developer friendly tools to make AI integration effortless. Our lightweight AI Rephrase library can be implemented in a few simple steps.
1. Import the QBAIRephrase module:
import QBAIRephrase
2. Rephrase text using the OpenAI API:
let text: String = ... // Replace with your text // Rephrase text using an API key do { let apiKey = "YOUR_OPENAI_API_KEY" let rephrasedText = try await QBAIRephrase.openAI(rephrase: text, tone: .empathetic, secret: apiKey) print("Rephrased Text: \(rephrasedText)") } catch { print("Error: \(error)") } // Rephrase text using a QuickBlox user token and proxy URL do { let qbToken = "YOUR_QUICKBLOX_USER_TOKEN" let proxyURL = "https://your-proxy-server-url" let rephrasedText = try await QBAIRephrase.openAI(rephrase: text, tone: .empathetic, qbToken: qbToken, proxy: proxyURL) print("Rephrased Text: \(rephrasedText)") } catch { print("Error: \(error)") }
Follow these steps to integrate QBAIRephrase into your project, and you’ll be able to rephrase text using the OpenAI API seamlessly.
We have already integrated the AI Rephrase feature within our iOS UI Kit. This feature leverages the OpenAI API key or proxy server to generate responses more securely and efficiently.
1. Open your project with QuickBlox UI Kit Swift package.
2. Configure the settings:
// Enable AI Rephrase QuickBloxUIKit.feature.ai.rephrase.enable = true
If this option is enabled, the menu bar for the Rephrase tones will appear above the chat message when you type the text.
When you click your selected tone, this message content will be rephrased.
Clicking “Back to original text” in a tone menu list will revert the text to its original version.
3. Set up the AI settings by providing either the OpenAI API key:
// Set OpenAI API key or proxy server URL QuickBloxUIKit.feature.ai.rephrase.apiKey = “YOUR_OPENAI_API_KEY”
Or set up with a proxy server:
QuickBloxUIKit.feature.ai.rephrase.serverPath = “https://your-proxy-server-url”
We recommend using a proxy server like the QuickBlox AI Assistant Proxy Server, which offers significant benefits in terms of security and functionality:
Developers using the AI Rephrase library have the ability to use Default Tones and Default AIRephraseSettings.
public class AIRephraseSettings { public var tones: [QBAIRephrase.AITone] = [ QBAIRephrase.AITone.professional, QBAIRephrase.AITone.friendly, QBAIRephrase.AITone.encouraging, QBAIRephrase.AITone.empathetic, QBAIRephrase.AITone.neutral, QBAIRephrase.AITone.assertive, QBAIRephrase.AITone.instructive, QBAIRephrase.AITone.persuasive, QBAIRephrase.AITone.sarcastic, QBAIRephrase.AITone.poetic ] /// Determines if assist answer functionality is enabled. public var enable: Bool = true /// The OpenAI API key for direct API requests (if not using a proxy server). public var apiKey: String = "" /// The URL path of the proxy server for more secure communication (if not using the API key directly). /// [QuickBlox AI Assistant Proxy Server](https://github.com/QuickBlox/qb-ai-assistant-proxy-server). public var serverPath: String = "" /// Represents the available API versions for OpenAI. public var apiVersion: QBAIRephrase.APIVersion = .v1 /// Optional organization information for OpenAI requests. public var organization: String? = nil /// Represents the available GPT models for OpenAI. public var model: QBAIRephrase.Model = .gpt3_5_turbo /// The temperature setting for generating responses (higher values make output more random). public var temperature: Float = 0.5 /// The maximum number of tokens to generate in the request. public var maxRequestTokens: Int = 3000 /// The maximum number of tokens to generate in the response. public var maxResponseTokens: Int? = nil }
Developers using the AI Rephrase library have the ability to delete tones, create and add their own tones to tailor the user interface to their needs. Also a developer has the ability to setup custom settings. Below is an example of creating custom tones and installing them in QuickBlox iOS UI Kit from the custom application.
import QBAIRephrase // Custom Tones public extension QBAIRephrase.AITone { static let youth = QBAIRephrase.AITone ( name: "Youth", description: "This will allow you to edit messages so that they sound youthful and less formal, using youth slang vocabulary that includes juvenile expressions, unclear sentence structure and without maintaining a formal tone. This will avoid formal speech and ensure appropriate youth greetings and signatures.", icon: "?" ) static let doctor = QBAIRephrase.AITone ( name: "Doctor", description: "This will allow you to edit messages so that they sound doctoral, using medical and medical vocabulary, including professional expressions, unclear sentence structure. This will allow you to make speeches in a medical-doctoral tone and provide appropriate medical greetings and signatures.", icon: "?" ) } // Array of required tones for your application. public var customTones: [QBAIRephrase.AITone] = [ .youth, // Custom Tone .doctor, // Custom Tone .sarcastic, // Default Tone .friendly, // Default Tone .empathetic, // Default Tone .neutral, // Default Tone .poetic // Default Tone ] // Setup an array of required tones for your application. QuickBloxUIKit.feature.ai.rephrase.tones = customTones // Setup custom settings for Rephrase. QuickBloxUIKit.feature.ai.rephrase.organization = "CustomDev" QuickBloxUIKit.feature.ai.rephrase.model = .gpt4 QuickBloxUIKit.feature.ai.rephrase.temperature = 0.8 QuickBloxUIKit.feature.ai.rephrase.maxRequestTokens = 3500
Additional examples of setting custom settings for the appearance of user interface elements from a user application can be found in our UIKitSample.
To use the OpenAI API for rephrasing, you need an API key. Follow these steps to get your API key:
Enhance security and functionality by using a proxy server like the QuickBlox AI Assistant Proxy Server
Integrating AI Rephrase into your iOS chat app has never been easier. By following the steps outlined above, you can seamlessly empower your users with real-time rephrasing capabilities using QuickBlox and the QBAIRephrase Swift package. Unlock the power of AI-driven interactions and enhance user communication in your app.
For more information and resources, explore the QuickBlox AI Proxy Server and the QBAIRephrase Swift package repositories.
Resources:
If you are interested in building additional AI-driven chat interfaces with AI check out other exciting AI features available with the iOS UI Kit, including AI Answer Assist and AI Translate.
– AI Translate Implementation Guide for iOS Apps
– AI Answer Assist Implementation Guide for iOS Apps
Planning to explore iOS chatbot development? Our new smartchat assistant feature, accessible via the QuickBout developer account dashboard, allows developers to build an AI-driven chatbot UX on iOS apps. It’s coming soon!
If you have any questions about AI-powered in-app chat for iOS please get in touch with our team!
Happy coding! ?