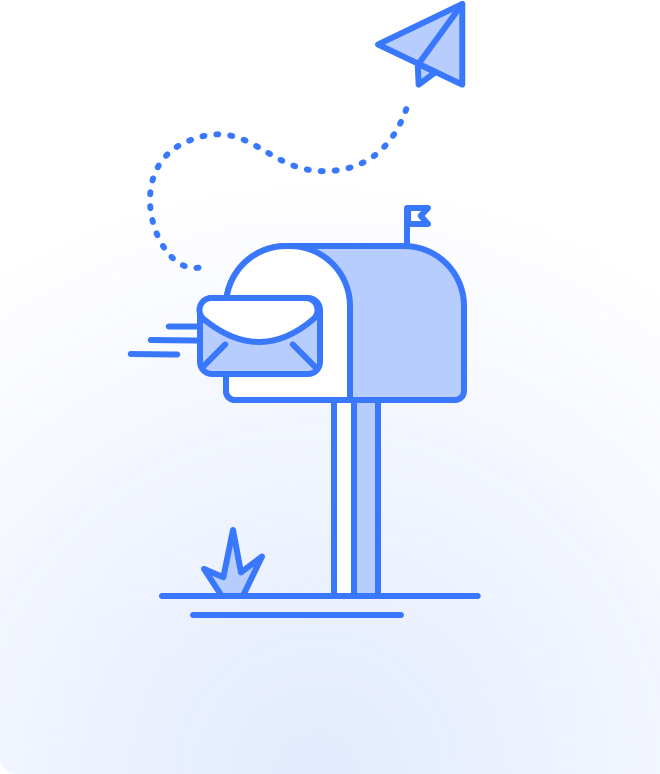
Welcome to a developer’s guide that unlocks a world of seamless cross-language communication within your iOS app. Imagine enabling users from different corners of the globe to connect effortlessly, breaking down language barriers in real-time.
In this tutorial, we’ll walk you through the process of integrating QuickBlox’s AI Translate feature, a cutting-edge tool that translates chat messages on-the-fly.
With QuickBlox, you can easily integrate AI Translate for iOS into your chat app. Whether you’re building a chat app from scratch or using our convenient iOS UI Kit, the QuickBlox iOS AI Library empowers you to seamlessly integrate AI translation capabilities using the QBAITranslate Swift package.
Learn more about: Getting Started with the QuickBlox iOS UI Kit
It’s easy to install the AI Translate library. Follow these simple few steps:
1. Import the QBAITranslate module:
import QBAITranslate
2. Set up your translation settings (by default used system language):
// Configure translation settings QBAITranslate.settings.setCustom(language: .spanish) // ... other settings
3. Call the `openAI` method to generate translations:
let text = ... do { let apiKey = "YOUR_OPENAI_API_KEY" let translation = try await QBAITranslate.openAI(translate: text, secret: apiKey) print("Generated Translation: \(translation)") } catch { print("Error: \(error)") }
We have already integrated the AI Translate feature within our iOS UI Kit. This feature leverages the OpenAI API key or proxy server to generate responses more securely and efficiently.
1. Open your project with QuickBlox UI Kit swift package.
2. Configure the settings:
// Enable AI Translate QuickBloxUIKit.feature.ai.translate.enable = true
If this option is enabled, the “Show translation” button will be displayed at the bottom of every incoming message in the chat interface.
When you click on “Show translation”, the chat message will be translated into the language set for translation.
A “Show original” button will be displayed. When clicked, the text of the message will immediately revert to the original version.
3. Set up the AI settings by providing either the OpenAI API key:
// Set OpenAI API key or proxy server URL QuickBloxUIKit.feature.ai.translate.apiKey = “YOUR_OPENAI_API_KEY
Or set up with a proxy server:
QuickBloxUIKit.feature.ai.translate.serverPath = “https://your-proxy-server-url”
We recommend using a proxy server like the QuickBlox AI Assistant Proxy Server which offers significant benefits in terms of security and functionality:
A developer using the AI Translate library has the ability to use Default QBAITranslate.Language and Default AITranslateSettings.
public class AITranslateSettings { /// The current `QBAITranslate.Language` /// /// Default the same as system language or `.english` if `QBAITranslate.Language` is not support system language. public var language: QBAITranslate.Language /// Determines if assist answer functionality is enabled. public var enable: Bool = true /// The OpenAI API key for direct API requests (if not using a proxy server). public var apiKey: String = "" /// The URL path of the proxy server for more secure communication (if not using the API key directly). /// [QuickBlox AI Assistant Proxy Server](https://github.com/QuickBlox/qb-ai-assistant-proxy-server). public var serverPath: String = "" /// Represents the available API versions for OpenAI. public var apiVersion: QBAITranslate.APIVersion = .v1 /// Optional organization information for OpenAI requests. public var organization: String? = nil /// Represents the available GPT models for OpenAI. public var model: QBAITranslate.Model = .gpt3_5_turbo /// The temperature setting for generating responses (higher values make output more random). public var temperature: Float = 0.5 /// The maximum number of tokens to generate in the request. public var maxRequestTokens: Int = 3000 /// The maximum number of tokens to generate in the response. public var maxResponseTokens: Int? = nil }
A developer using the AI Translate library has the ability to setup custom translation language (by default used system language). Also a developer has the ability to setup custom settings. Below is an example of creating custom tones and installing them in QuickBlox iOS UI Kit from the custom application.
import QBAITranslate // Set up the language for translation(by default used system language) QuickBloxUIKit.feature.ai.translate.language = .spanish // Setup custom settings for Translate. QuickBloxUIKit.feature.ai.translate.organization = "CustomDev" QuickBloxUIKit.feature.ai.translate.model = .gpt4 QuickBloxUIKit.feature.ai.translate.temperature = 0.8 QuickBloxUIKit.feature.ai.translate.maxRequestTokens = 3500
Additional examples of setting custom settings for the appearance of user interface elements from a user application can be found in our UIKitSample.
To use the OpenAI API for translation, you need an API key. Follow these steps to get your API key:
1. Sign in to your OpenAI account or create a new one on the OpenAI website.
2. Navigate to the API section and sign up for API access.
3. Once approved, obtain your API key.
Enhance security and functionality by using a proxy server like the QuickBlox AI Assistant Proxy Server so that you can enjoy the following benefits:
– Protect sensitive information like API keys.
– Implement access control mechanisms for secure communication.
– Mitigate the risk of API key exposure.
– Enforce rate limiting and throttling for API usage compliance.
– Monitor and log API requests for auditing purposes.
If you are looking to build an Android translation app, harnessing the power of artificial intelligence for language translation, then you need to consider using QuickBlox and the QBAITranslate Swift package.
By following our tutorial for iOS app development with AI translation, you can seamlessly empower your app users with real-time translation capabilities.
Integrating AI Translate into your iOS chat app allows you to unlock the power of AI-driven interactions and enhance user communication.
For more information and resources, explore the QuickBlox AI Assistant Proxy Server and the QBAITranslate Swift package repositories.
– QBAIProxy Server
– QBAITranslate Swift Package
– QuickBlox Documentation
Happy coding! ?