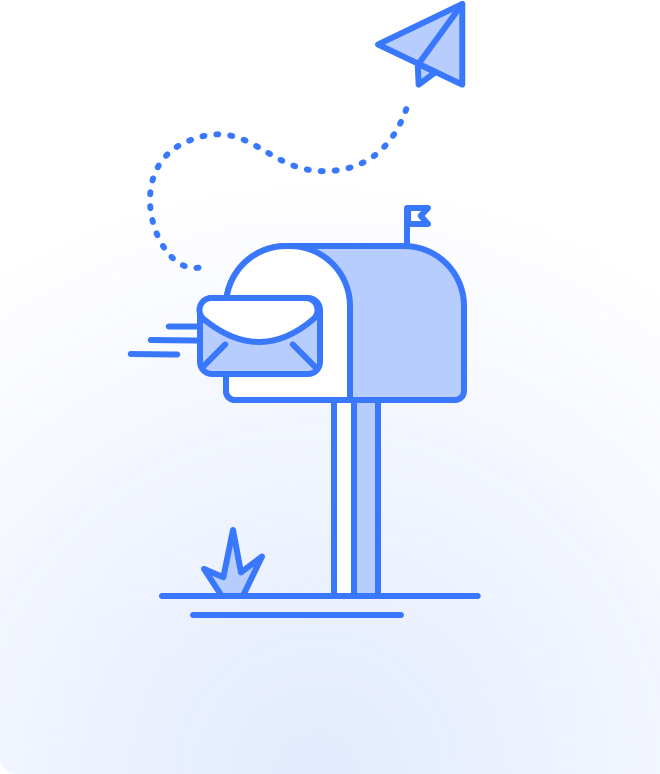
Adding AI functionality to real-time communication applications is crucial for creating unique and innovative products. QuickBlox aims to make this process easy for developers by offering advanced AI libraries for integration into JavaScript applications.
In the following two tutorials we will guide you through the process of integrating three key AI functions — Answer Assist, Translate, and Rephrase — into your NodeJS application. Using GPT OpenAI technology, we provide you with a unique opportunity to enhance your application’s communication capabilities, providing quick and contextually relevant answers to user queries, easily overcoming language barriers, and rephrasing the tone of text messages with a click of a button.
In this first article, we will walk you through creating a client-server application in NodeJS using the Express framework. But watch out for part 2 where we’ll present you with a practical example of integrating QuickBlox’s AI libraries, helping you better understand and use them in your own project.
Let’s work together to build an incredible application where the power AI becomes an integral part of your app’s functionality.
Let’s jump into the practical side of things and set up our NodeJS application using the Express framework. Follow these simple steps to create a solid foundation for integrating QuickBlox’s AI libraries.
Create a new directory for your project and navigate into it using the terminal. Run the following commands to initialize your NodeJS application:
npm init -y
This will generate a package.json file with default settings.
Ensure you have Node.js installed on your machine. If not, download and install it from nodejs.org. Once Node.js is ready, install Express by running the following command in your terminal:
npm install express --save
Nodemon is a helpful tool for automatically restarting your server when changes are detected. Install it by running:
npm install nodemon --save-dev
Open your package.json file and add the following scripts to enable easy startup with Nodemon:
"scripts": { "run": "node app", "dev": "nodemon app" },
Now, create an entry file, let’s call it app.js. In this file, import Express and set up a basic server:
const express = require('express'); const bodyParser = require('body-parser'); const exphbs = require('express-handlebars'); const app = express(); const port = 3000; // Use Body Parser middleware app.use(bodyParser.urlencoded({ extended: false })); app.use(bodyParser.json()); // Set up Handlebars as the view engine app.engine('handlebars', exphbs()); app.set('view engine', 'handlebars'); // Basic route app.get('/', (req, res) => { res.send('Hello, World!'); }); app.listen(port, () => { console.log(`Server is running at http://localhost:${port}`); });
Body-parser simplifies the process of handling HTTP POST requests, and Express Handlebars is a view engine for Express. Install them using:
npm install body-parser express-handlebars --save
Run your server by executing:
npm start
Visit http://localhost:3000 in your web browser, and you should see “Hello, World!”.
To enhance the templating capabilities of our NodeJS application, we’ll modify the project structure to accommodate Handlebars. Follow these steps to create a more organized layout for views using Express Handlebars.
In your project, create a new directory named views. Inside this directory, set up the following subdirectories and files as illustrated in the screenshot:
- views - layouts - main.hbs - partials - head.hbs - navbar.hbs - footer.hbs - index.hbs - assist.hbs - translate.hbs - rephrase.hbs
Open the main.hbs file inside the layouts directory and structure it as the main template for your pages. Add partials for the head, navbar, and footer by creating the corresponding head.hbs, navbar.hbs, and footer.hbs files inside the partials directory.
In your app.js file, make the following changes to configure Express Handlebars:
const exphbs = require('express-handlebars'); const path = require('path'); // Set up Handlebars as the view engine const hbs = exphbs.create({ defaultLayout: 'main', extname: 'hbs', layoutsDir: path.join(__dirname, 'views', 'layouts'), partialsDir: path.join(__dirname, 'views', 'layouts', 'partials') }); app.engine('hbs', hbs.engine); app.set('view engine', 'hbs'); app.set('views', 'views');
To further organize our project, we’ll introduce three controllers in the controllers directory: assistController.js, translateController.js, and rephraseController.js. These controllers will encapsulate the logic for working with QuickBlox’s AI libraries.
In your project, create a new directory named controllers. Inside this directory, add the following files:
- controllers - assistController.js - translateController.js - rephraseController.js
In assistController.js:
// assistController.js module.exports.aiCreateAnswer = async function (req, res) { res.render('assist', { title: 'AI Assist', isAssist: true }); } module.exports.showFormAssist = async function (req, res) { res.render('assist', { title: 'AI Assist', isAssist: true }); }
In translateController.js:
// translateController.js module.exports.aiCreateTranslate = async function (req, res) { res.render('translate', { title: 'AI Translate', isTranslate: true }); } module.exports.showFormTranslate = async function (req, res) { res.render('translate', { title: 'AI Translate', isTranslate: true, history: JSON.stringify(history, null, 4), textToTranslate, }); }
In rephraseController.js:
// rephraseController.js module.exports.aiCreateRephrase = async function (req, res) { res.render('rephrase', { title: 'AI Rephrase', isRephrase: true }); } module.exports.showFormRephrase = async function (req, res) { res.render('rephrase', { title: 'AI Rephrase', isRephrase: true }); }
To enhance the structure of our project, we’ll introduce four routers in the routes directory:defaultRoute.js, aiAssistRoute.js, aiTranslateRoute.js, and aiRephraseRoute.js. These routers will invoke functionalities from the controllers.
In your project, create a new directory named routes. Inside this directory, add the following files:
- routes - defaultRoute.js - aiAssistRoute.js - aiTranslateRoute.js - aiRephraseRoute.js
In defaultRoute.js:
// defaultRoute.js const { Router } = require('express'); const router = Router(); router.get('/', (req, res) => { res.render('index', { title: 'QuickBlox AI libraries', isIndex: true }); }); module.exports = router;
In aiAssistRoute.js:
// aiAssistRoute.js const { Router } = require('express'); const assistController = require('../controllers/assistController'); const router = Router(); router.get('/', assistController.showFormAssist); router.post('/', assistController.aiCreateAnswer); module.exports = router;
In aiTranslateRoute.js:
// aiTranslateRoute.js const { Router } = require('express'); const translateController = require('../controllers/translateController'); const router = Router(); router.get('/', translateController.showFormTranslate); router.post('/', translateController.aiCreateTranslate); module.exports = router;
In aiRephraseRoute.js:
// aiRephraseRoute.js const { Router } = require('express'); const rephraseController = require('../controllers/rephraseController'); const router = Router(); router.get('/', rephraseController.showFormRephrase); router.post('/', rephraseController.aiCreateRephrase); module.exports = router;
In your app.js file, update the route handlers to utilize the new routers:
const defaultRoute = require('./routes/defaultRoute'); const aiAssistRoute = require('./routes/aiAssistRoute'); const aiTranslateRoute = require('./routes/aiTranslateRoute'); const aiRephraseRoute = require('./routes/aiRephraseRoute'); // Use routers app.use('/', defaultRoute); app.use('/assist', aiAssistRoute); app.use('/translate', aiTranslateRoute); app.use('/rephrase', aiRephraseRoute);
With these changes, you’ve refined your project structure by introducing routers to manage the routes. Each router invokes the corresponding functionality from the controllers. This modular approach makes your code more maintainable and easier to understand.
Congratulations! You’ve successfully set up a basic NodeJS application with Express and Nodemon, laying the groundwork for integrating QuickBlox’s AI libraries.
In part two of this article we’ll explore how to incorporate Answer Assist, Translate, and Rephrase functionalities seamlessly into your application.
Whether you seek more detailed information or are ready to dive into experimenting with these libraries, the source code of the application featured in the article is available in the QuickBlox GitHub Repository. Enjoy coding and exploring the possibilities of artificial intelligence in your projects!